Gradio: your first web application#
In this tutorial, you will learn how to build your first Gradio web application. To be able to create a Gradio application, you will first need to configure a Code Studio template and then code your application using this application. Once the application is designed, you can publish it as a web application.
Prerequisites#
Administrator permission to build the template
An LLM connection configured
Building the Code Studio template#
Go to the Code Studios tab in the Administration menu, click the +Create Code Studio template button, and choose a meaningful label (
gradio_template
, for example).Click on the Definition tab.
Add a new Visual Studio Code block. This block will allow you to edit your Gradio application in a dedicated Code Studio.
Add a new Gradio block, and choose Generate a dedicated code env for the Code env mode, as shown in Figure 1.
Click the Save button.
Click the Build button to build the template.
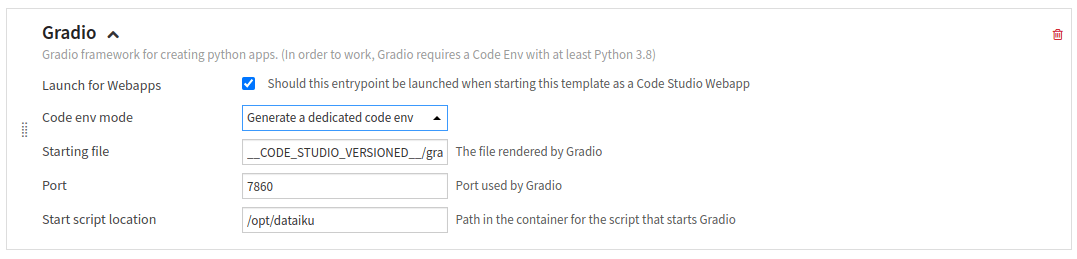
Figure 1: Code Studio – Gradio block.#
Your Code Studio template is ready to be used in a project. If you need a specific configuration for a block, please refer to the documentation. If you want to generate your code environment to include other packages than Gradio, you should pin the Gradio version to 3.48.0.
Creating a new Gradio application#
Before creating your Gradio application, be sure to have a valid LLM ID. You can refer to the documentation to retrieve an LLM ID.
Create a new project, click on </> > Code Studios.
Click the +New Code Studio, choose the previously created template, choose a meaningful name, click the Create button, and then click the Start Code Studio button.
To edit the code of your Gradio application, click the highlighted tabs (VS Code) as shown in Figure 2.
Select the
gradio
subdirectory in thecode_studio-versioned
directory. Dataiku provides a sample application in the fileapp.py
.Replace the provided code with the code shown in Code 1. Replace the LLM_ID with your LLM_ID in the highlighted line.
If everything goes well, you should have a running Gradio application like the one shown in Figure 3.

Figure 2: Code Studio.#
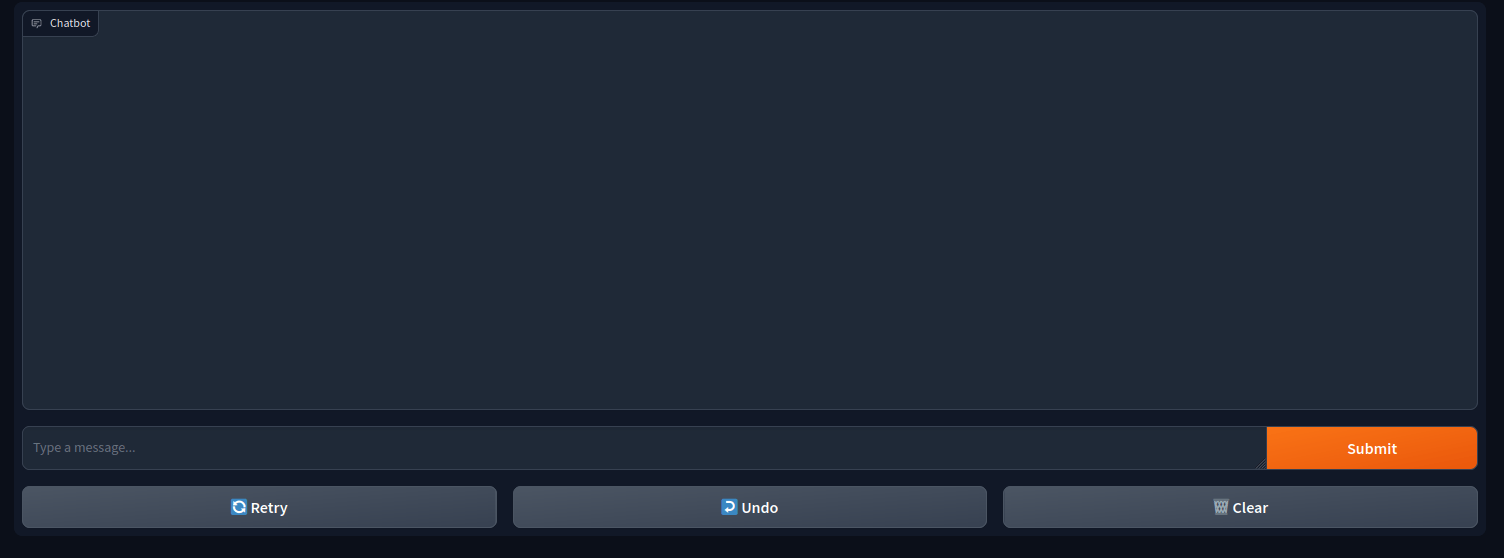
Figure 3: First Gradio application using a LLM.#
Wrapping up#
You now have a running Gradio application. You can customize it a little to fit your needs. When you are happy with the result, you can click on the Publish button in the right panel. Then, your Gradio application is available for all users who can use it without running the Code Studio.
app.py
import gradio as gr
import os
import re
import dataiku
LLM_ID = "YOUR_LLM_ID"
browser_path = os.getenv("DKU_CODE_STUDIO_BROWSER_PATH_7860")
env_var_pattern = re.compile(r'(\${(.*)})')
env_vars = env_var_pattern.findall(browser_path)
for env_var in env_vars:
browser_path = browser_path.replace(env_var[0], os.getenv(env_var[1], ''))
client = dataiku.api_client()
project = client.get_default_project()
llm = project.get_llm(LLM_ID)
def chat_function(message, history):
completion = llm.new_completion()
completion.with_message(message="You are a helpful assistant.")
for msg in history:
completion.with_message(message=msg[0], role="user")
completion.with_message(message=msg[1], role="assistant")
completion.with_message(message=message, role="user")
resp_text = completion.execute().text
return resp_text
demo = gr.ChatInterface(fn=chat_function)
demo.launch(server_port=7860, root_path=browser_path)